Learn Object Pascal
Part 3 - Data types, constants and variables
Let's see some basic data types.String data types:
char : A char is a single character.
ShortString : Short strings have a maximum length of 255 characters
String : If it is on ({$H+}) string without length specifier will define an AnsiString (default), otherwise if the switch is off ({$H-}) then any string declaration will define a ShortString.
Integer data types:
One bit can have two states 0 and 1.
Two bits can define four states (00,01,10,11).
Three bits can define eight states (000,001,010,011,100,101,110,111) and so on...
So with n bits we can define 2^n_bits different states.
A byte contain 8 bits.
So Max range = (2^(8*Bytes))-1 for unsigned integer types
byte range: 0 .. 255 Bytes: 1 unsigned
Shortint range: -128 .. 127 Bytes: 1 signed
Word range: 0 .. 65535 Bytes: 2 unsigned
Smallint range: -32768 .. 32767 Bytes: 2 signed
Cardinal range: 0..4294967295 Bytes: 4 unsigned
Longword range: 0..4294967295 Bytes: 4 unsigned
Integer range: -2147483648 .. 2147483647 Bytes: 4 signed
Longint range: -2147483648 .. 2147483647 Bytes: 4 signed
QWord range: 0 .. 18446744073709551615 Bytes: 8 unsigned
Real data types:
Real data types follow the IEEE floating point types.
The IEEE 754 single-precision binary floating occupies 4 bytes (32 bits) and is defined like this that :
[sign 1 bit][exponent 8 bits][fraction or mantissa 23 bits]
The formula that gives the value of the floating point is :
value = (-1)^sign * ( 1 + fraction_1_bit*2^-1 + fraction_2_bit*2^-2 + ... + fraction_23_bit*2^-23 ) * 2^(exponent_base_10-127)
Example : 0 10000000 1 1 1 00000000000000000001
(-1)^0 * ( 1 + 1*2^-1 + 1*2^-2 + 1*2^-3 + 0*2^-4 + ... + 0*2^-22 + 1*2^-23 ) * 2^(128-127) = 3.7500002384185
The IEEE 754 double-precision binary floating occupies 8 bytes (64 bits) and is defined like this that :
[sign 1 bit][exponent 11 bits][fraction or mantissa 52 bits]
The formula that gives the value of the floating point is :
value = (-1)^sign * ( 1 + fraction_1_bit*2^-1 + fraction_2_bit*2^-2 + ... + fraction_52_bit*2^-52 ) * 2^(exponent_base_10-1023)
In Pascal we have these real data types :
Real range: n/a Significant digits: n/a Bytes: 4 or 8
Single range: 1.5E-45 .. 3.4E38 Significant digits: 7-8 Bytes: 4
Double range: 5.0E-324 .. 1.7E308 Significant digits: 15-16 Bytes: 8
Extended range: 1.9E-4932 .. 1.1E4932 Significant digits: 19-20 Bytes: 10
Comp range: -2E64+1 .. 2E63-1 Significant digits: 19-20 Bytes: 8
Currency range: -922337203685477.5808 922337203685477.5807 Significant digits:19-20 Bytes: 8
Boolean data types:
Boolean 1 byte
These are all the basic data types.
A variable is a name given to a storage area that our programs can read and change.
Let's see how to define / declare some variables using a specific data type.
var
counter, age : integer;
isOld : boolean;
Notice that variables with the same data type must be separated with commas. You can also separate the variables but it is not recommended (see below):
var
counter : integer;
age : integer;
isOld : boolean;
Variables can be assigned like this :
counter:=0;
age:=20;
isOld:=false;
Variables can be initialized (assigned an initial value) in their declaration like this :
var
counter : integer = 10;
age : integer = 70;
isOld : boolean = true;
After the initialization we can reassign the variables as we like during the program.
counter:=100;
A constant is a name given to a storage area that our programs can only read. Constants can be assigned one value at the beginning of the program.
The value stored in a constant cannot be changed.
Constants may contain a datatype.
const
PI = 3.141592654; //constant declaration
a : integer = 33; {typed constant declaration
that force a constant to be of a particular data type.}
Let's make a demo program using variables and constants.
Using 'File'>'New'>'Application' create a new empty project.
Now design a Button and a Memo.
At the Memo change the property 'ScrollBars' to "ssAutoBoth" value.
By doing this we will have scrollbars into our Memo1 component.
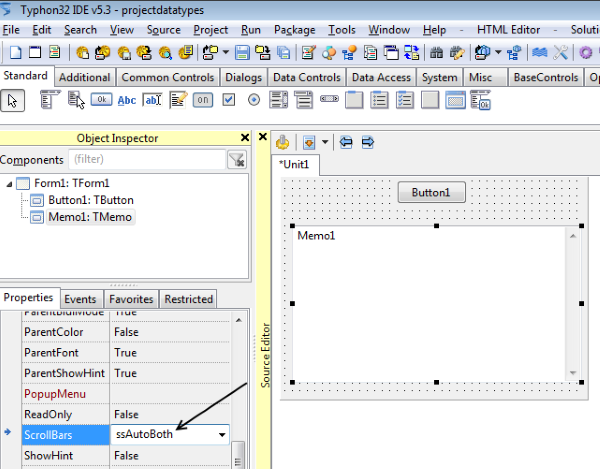
At the OnClick event of the Button1 write
procedure TForm1.Button1Click(Sender: TObject);
const
PI = 3.141592654; //constant declaraion
a : integer = 33; {typed constant declaration that
force a constant to be of a particular data type.}
var
//String types :
achar : char; //A char is a single character.
aShortString : ShortString; //Short strings have a maximum length of 255 characters
aString : String; {If it is on $H+ string without length specifier
will define an AnsiString (default), otherwise if the switch is off $H- then any
string declaration will define a ShortString. }
//Integer Types :
//Max rage = (2^(8*Bytes))-1 for unsigned types
abyte : byte; //0 .. 255 Bytes : 1 unsigned
aShortint : Shortint; //-128 .. 127 Bytes : 1 signed
aWord : Word; //0 .. 65535 Bytes : 2 unsigned
aSmallint : Smallint; //-32768 .. 32767 Bytes : 2 signed
aCardinal : Cardinal; //0..4294967295 Bytes : 4 unsigned
aLongword : Longword; //0..4294967295 Bytes : 4 unsigned
aInteger : Integer; //-2147483648 .. 2147483647 Bytes : 4 signed
aLongint : Longint; //-2147483648 .. 2147483647 Bytes : 4 signed
aQWord : QWord; //0 .. 18446744073709551615 Bytes : 8 unsigned
aInt64 : Int64; //-9223372036854775808 .. 9223372036854775807 Bytes : 8 signed
//Real Type :
aSingle : Single;
aDouble : Double;
//Boolean :
aBoolean,bBoolean : Boolean;
//Extra
counter, age : integer;
isOld : boolean = true; //initialize this variable to true
begin
//Assign constants NOT ALLOWED
//PI := 3; //THIS IS INCORRECT You can not assign a constant
//Assign variables
achar:='C';
aShortString:='Short string';
aString:='String normal';
abyte:=255;
aShortint:=127;
aWord:=65535;
aSmallint:=32767;
aCardinal:=4294967295;
aLongword:=4294967295;
aInteger:=2147483647;
aLongint:=2147483647;
aQWord:=18446744073709551615;
aInt64:=9223372036854775807;
aSingle:=1.75;
aDouble:=2.5E100; //2.5^100
aBoolean:=true;
bBoolean:=false;
counter:=0;
age:=20;
isOld:=false;
//print variables
Memo1.Lines.Add(achar);
Memo1.Lines.Add(aShortString);
Memo1.Lines.Add(aString);
Memo1.Lines.Add(IntToStr(abyte)); //IntToStr : convert integer into string
Memo1.Lines.Add(IntToStr(aShortint));
Memo1.Lines.Add(IntToStr(aWord));
Memo1.Lines.Add(IntToStr(aSmallint));
Memo1.Lines.Add(IntToStr(aCardinal));
Memo1.Lines.Add(IntToStr(aLongword));
Memo1.Lines.Add(IntToStr(aInteger));
Memo1.Lines.Add(IntToStr(aLongint));
Memo1.Lines.Add(IntToStr(aQWord));
Memo1.Lines.Add(IntToStr(aInt64));
Memo1.Lines.Add(FloatToStr(aSingle)); //FloatToStr : convert float into string
Memo1.Lines.Add(FloatToStr(aDouble));
Memo1.Lines.Add(BoolToStr(aBoolean)); //-1 : true //BoolToStr : convert boolean into string
Memo1.Lines.Add(BoolToStr(bBoolean)); // 0 : false
Memo1.Lines.Add('Counter: ' + IntToStr(counter)); //string concatenation
Memo1.Lines.Add('Age: ' + IntToStr(age)); //string concatenation
Memo1.Lines.Add(BoolToStr(isOld)); //-1 : true
Memo1.Lines.Add('PI: ' + FloatToStr(PI)); //string concatenation
Memo1.Lines.Add('a integer constant: ' + IntToStr(a)); //string concatenation
end;
Notice that the function :
Memo1.Lines.Add('YOURSTRING');
adds a line into the Memo1 component. The add function need a string as input that's why we are using IntToStr for integers since this function convert the integer into a string.
BoolToStr and FloatToStr do the same for booleans and floats respectively
Also notice that we can concatenate strings using the plus (+) symbol (operator).
If you compile the program by pressing the Button1 you will be able to see all the values of your constants and variables into the Memo component.
This Datatypes - GUI project can be downloaded from here.
In the next session we will talk about operators.
Part 1: Introduction - Part 2: Hello World - [[ Part 3: Data types, constants and variables ]] - Part 4: Operators - Part 5: Decisions and Loops - Part 6: Procedures and Functions - Part 7: Custom datatypes - Part 8: Enumerations, subranges and sets - Part 9: Records